OpnePyXLでエクセルのフォント設定をします。
サンプルデータとして、エクセルに文字を書き、ファイル出力します。
import os import openpyxl wb = openpyxl.Workbook() ws = wb.active ws.cell(row=1, column=1).value = 'あいうえお' ws.cell(row=2, column=1).value = 'カキクケコ' ws.cell(row=3, column=1).value = '日本東京都' ws.cell(row=4, column=1).value = '123456789' ws.cell(row=5, column=1).value = 'This is a pen.' filename = 'test_a.xlsx' file_path = os.path.join(os.getcwd(), filename) wb.save(file_path)
このようなエクセルが出力されます。
デフォルトの文字の種類はMS Pゴシックになっています。
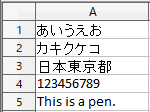
文字の種類を設定する
文字の種類を設定します。
openpyxl.styles.fonts のドキュメントは こちら です。
文字の種類の設定は “name” パラメータです。
“name : Values must be of type ” となっているので、Str型で設定します。
Font(name=”MS P明朝”) のように、文字列で指定します。
エクセルを配布する場合は、配布先でも使える文字を指定すべきで、エクセルにデフォルトで入っている文字を指定した方が良さそうです。
下の例では、name=”游ゴシック Medium” を指定しています。
わかりやすいように、”bold” パラメータも指定します。
import os import openpyxl from openpyxl.styles import Font wb = openpyxl.Workbook() ws = wb.active ws.cell(row=1, column=1).value = 'あいうえお' ws.cell(row=2, column=1).value = 'カキクケコ' ws.cell(row=3, column=1).value = '日本東京都' ws.cell(row=4, column=1).value = '123456789' ws.cell(row=5, column=1).value = 'This is a pen.' font = Font(name='遊ゴシック Medium', bold=True) for row in range(1, ws.max_row + 1): for col in range(1, ws.max_column + 1): ws.cell(row=row, column=col).font = font filename = 'test_b.xlsx' file_path = os.path.join(os.getcwd(), filename) wb.save(file_path)
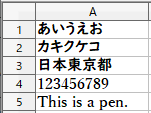
複数のワークシートにまたがって、文字を設定する
実際の現場では複数シートある場合が多いので、複数シートにまたがる場合もやってみます。
import os import openpyxl from openpyxl.styles import Font wb = openpyxl.Workbook() ws = wb.active ws.cell(row=1, column=1).value = 'あいうえお' ws.cell(row=2, column=1).value = 'カキクケコ' ws.cell(row=3, column=1).value = '日本東京都' ws.cell(row=4, column=1).value = '123456789' ws.cell(row=5, column=1).value = 'This is a pen.' ws = wb.create_sheet('test') # "test"という名前のワークシートを作成する。 ws.cell(row=1, column=1).value = 'さしすせそ' # ここまでで、"Sheet"と"test"という2つのワークシートが存在するので、 # その2つのワークシートの文字の設定を設定する。 font = Font(name='メイリオ', size=9) # フォント設定 for ws_name in wb.sheetnames: # ワークブックに存在するワークシート名でFor文を回す。 ws = wb[ws_name] for row in range(1, ws.max_row + 1): for col in range(1, ws.max_column + 1): ws.cell(row=row, column=col).font = font filename = 'test_c.xlsx' file_path = os.path.join(os.getcwd(), filename) wb.save(file_path)